Commit f98ac33089a8879d6f28def93745548ba8549839
Committed by
Michel Felipe
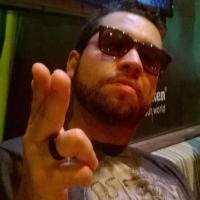
1 parent
7b6978a6
Exists in
master
and in
26 other branches
Add tests for comment paragraph plugin
Showing
10 changed files
with
366 additions
and
12 deletions
Show diff stats
src/app/hotspot/hotspot.decorator.ts
src/plugins/comment_paragraph/allow-comment/allow-comment.component.spec.ts
0 → 100644
... | ... | @@ -0,0 +1,78 @@ |
1 | +import {AllowCommentComponent} from "./allow-comment.component"; | |
2 | +import {ComponentTestHelper, createClass} from '../../../spec/component-test-helper'; | |
3 | +import * as helpers from "../../../spec/helpers"; | |
4 | +import {Provider} from 'ng-forward'; | |
5 | +import {ComponentFixture} from 'ng-forward/cjs/testing/test-component-builder'; | |
6 | + | |
7 | +let htmlTemplate = '<comment-paragraph-plugin-allow-comment [content]="ctrl.content" [paragraph-uuid]="ctrl.paragraphUuid" [article]="ctrl.article"></comment-paragraph-plugin-allow-comment>'; | |
8 | + | |
9 | +describe("Components", () => { | |
10 | + describe("Allow Comment Component", () => { | |
11 | + | |
12 | + let serviceMock = { | |
13 | + commentParagraphCount: () => { | |
14 | + return Promise.resolve(5); | |
15 | + } | |
16 | + }; | |
17 | + let functionToggleCommentParagraph: Function; | |
18 | + let eventServiceMock = { | |
19 | + // toggleCommentParagraph | |
20 | + subscribeToggleCommentParagraph: (fn: Function) => { | |
21 | + functionToggleCommentParagraph = fn; | |
22 | + } | |
23 | + }; | |
24 | + | |
25 | + let providers = [ | |
26 | + new Provider('CommentParagraphService', { useValue: serviceMock }), | |
27 | + new Provider('CommentParagraphEventService', { useValue: eventServiceMock }) | |
28 | + ]; | |
29 | + let helper: ComponentTestHelper; | |
30 | + | |
31 | + beforeEach(angular.mock.module("templates")); | |
32 | + | |
33 | + beforeEach((done) => { | |
34 | + let cls = createClass({ | |
35 | + template: htmlTemplate, | |
36 | + directives: [AllowCommentComponent], | |
37 | + providers: providers, | |
38 | + properties: { | |
39 | + content: "", | |
40 | + paragraphUuid: "uuid", | |
41 | + article: { | |
42 | + setting: { | |
43 | + comment_paragraph_plugin_activate: true | |
44 | + } | |
45 | + } | |
46 | + } | |
47 | + }); | |
48 | + helper = new ComponentTestHelper(cls, done); | |
49 | + }); | |
50 | + | |
51 | + it('update comments count', () => { | |
52 | + expect(helper.component.commentsCount).toEqual(5); | |
53 | + }); | |
54 | + | |
55 | + it('display paragraph content', () => { | |
56 | + expect(helper.all(".paragraph .paragraph-content").length).toEqual(1); | |
57 | + }); | |
58 | + | |
59 | + it('display button to side comments', () => { | |
60 | + expect(helper.all(".paragraph .actions a").length).toEqual(1); | |
61 | + }); | |
62 | + | |
63 | + it('set display to true when click in show paragraph', () => { | |
64 | + helper.component.showParagraphComments(); | |
65 | + expect(helper.component.display).toBeTruthy(); | |
66 | + }); | |
67 | + | |
68 | + it('set display to false when click in hide paragraph', () => { | |
69 | + helper.component.hideParagraphComments(); | |
70 | + expect(helper.component.display).toBeFalsy(); | |
71 | + }); | |
72 | + | |
73 | + it('update article when receive a toogle paragraph event', () => { | |
74 | + functionToggleCommentParagraph({ id: 2 }); | |
75 | + expect(helper.component.article.id).toEqual(2); | |
76 | + }); | |
77 | + }); | |
78 | +}); | ... | ... |
src/plugins/comment_paragraph/allow-comment/allow-comment.component.ts
... | ... | @@ -26,13 +26,13 @@ export class AllowCommentComponent { |
26 | 26 | this.article = article; |
27 | 27 | this.$scope.$apply(); |
28 | 28 | }); |
29 | - this.commentParagraphService.commentParagraphCount(this.article, this.paragraphUuid).then((count: any) => { | |
29 | + this.commentParagraphService.commentParagraphCount(this.article, this.paragraphUuid).then((count: number) => { | |
30 | 30 | this.commentsCount = count; |
31 | 31 | }); |
32 | 32 | } |
33 | 33 | |
34 | 34 | isActivated() { |
35 | - return this.article.setting.comment_paragraph_plugin_activate; | |
35 | + return this.article && this.article.setting && this.article.setting.comment_paragraph_plugin_activate; | |
36 | 36 | } |
37 | 37 | |
38 | 38 | showParagraphComments() { | ... | ... |
src/plugins/comment_paragraph/events/comment-paragraph-event.service.spec.ts
0 → 100644
... | ... | @@ -0,0 +1,28 @@ |
1 | +import {CommentParagraphEventService} from "./comment-paragraph-event.service"; | |
2 | +import {ComponentTestHelper, createClass} from '../../../spec/component-test-helper'; | |
3 | +import * as helpers from "../../../spec/helpers"; | |
4 | +import {Provider} from 'ng-forward'; | |
5 | +import {ComponentFixture} from 'ng-forward/cjs/testing/test-component-builder'; | |
6 | + | |
7 | +describe("Services", () => { | |
8 | + describe("Comment Paragraph Event Service", () => { | |
9 | + let eventService: CommentParagraphEventService; | |
10 | + | |
11 | + beforeEach(() => { | |
12 | + eventService = new CommentParagraphEventService(); | |
13 | + eventService['toggleCommentParagraphEmitter'] = jasmine.createSpyObj("toggleCommentParagraphEmitter", ["next", "subscribe"]); | |
14 | + }); | |
15 | + | |
16 | + it('subscribe to toggle comment paragraph event', () => { | |
17 | + eventService['toggleCommentParagraphEmitter'].subscribe = jasmine.createSpy("subscribe"); | |
18 | + eventService.subscribeToggleCommentParagraph(() => { }); | |
19 | + expect(eventService['toggleCommentParagraphEmitter'].subscribe).toHaveBeenCalled(); | |
20 | + }); | |
21 | + | |
22 | + it('emit event when toggle comment paragraph', () => { | |
23 | + eventService['toggleCommentParagraphEmitter'].subscribe = jasmine.createSpy("next"); | |
24 | + eventService.toggleCommentParagraph(<noosfero.Article>{}); | |
25 | + expect(eventService['toggleCommentParagraphEmitter'].next).toHaveBeenCalled(); | |
26 | + }); | |
27 | + }); | |
28 | +}); | ... | ... |
src/plugins/comment_paragraph/hotspot/comment-paragraph-article-button.component.spec.ts
0 → 100644
... | ... | @@ -0,0 +1,85 @@ |
1 | +import {CommentParagraphArticleButtonHotspotComponent} from "./comment-paragraph-article-button.component"; | |
2 | +import {ComponentTestHelper, createClass} from '../../../spec/component-test-helper'; | |
3 | +import * as helpers from "../../../spec/helpers"; | |
4 | +import {Provider} from 'ng-forward'; | |
5 | +import {ComponentFixture} from 'ng-forward/cjs/testing/test-component-builder'; | |
6 | + | |
7 | +let htmlTemplate = '<comment-paragraph-article-button-hotspot [article]="ctrl.article"></comment-paragraph-article-button-hotspot>'; | |
8 | + | |
9 | +describe("Components", () => { | |
10 | + describe("Comment Paragraph Article Button Hotspot Component", () => { | |
11 | + | |
12 | + let serviceMock = jasmine.createSpyObj("CommentParagraphService", ["deactivateCommentParagraph", "activateCommentParagraph"]); | |
13 | + let eventServiceMock = jasmine.createSpyObj("CommentParagraphEventService", ["toggleCommentParagraph"]); | |
14 | + | |
15 | + let providers = [ | |
16 | + new Provider('CommentParagraphService', { useValue: serviceMock }), | |
17 | + new Provider('CommentParagraphEventService', { useValue: eventServiceMock }) | |
18 | + ].concat(helpers.provideFilters('translateFilter')); | |
19 | + let helper: ComponentTestHelper; | |
20 | + | |
21 | + beforeEach(angular.mock.module("templates")); | |
22 | + | |
23 | + beforeEach((done) => { | |
24 | + let cls = createClass({ | |
25 | + template: htmlTemplate, | |
26 | + directives: [CommentParagraphArticleButtonHotspotComponent], | |
27 | + providers: providers, | |
28 | + properties: { | |
29 | + article: {} | |
30 | + } | |
31 | + }); | |
32 | + helper = new ComponentTestHelper(cls, done); | |
33 | + }); | |
34 | + | |
35 | + it('emit event when deactivate comment paragraph in an article', () => { | |
36 | + serviceMock.deactivateCommentParagraph = jasmine.createSpy("deactivateCommentParagraph").and.returnValue( | |
37 | + { then: (fn: Function) => { fn({ data: {} }); } } | |
38 | + ); | |
39 | + eventServiceMock.toggleCommentParagraph = jasmine.createSpy("toggleCommentParagraph"); | |
40 | + helper.component.deactivateCommentParagraph(); | |
41 | + | |
42 | + expect(serviceMock.deactivateCommentParagraph).toHaveBeenCalled(); | |
43 | + expect(eventServiceMock.toggleCommentParagraph).toHaveBeenCalled(); | |
44 | + }); | |
45 | + | |
46 | + it('emit event when activate comment paragraph in an article', () => { | |
47 | + serviceMock.activateCommentParagraph = jasmine.createSpy("activateCommentParagraph").and.returnValue( | |
48 | + { then: (fn: Function) => { fn({ data: {} }); } } | |
49 | + ); | |
50 | + eventServiceMock.toggleCommentParagraph = jasmine.createSpy("toggleCommentParagraph"); | |
51 | + helper.component.activateCommentParagraph(); | |
52 | + | |
53 | + expect(serviceMock.activateCommentParagraph).toHaveBeenCalled(); | |
54 | + expect(eventServiceMock.toggleCommentParagraph).toHaveBeenCalled(); | |
55 | + }); | |
56 | + | |
57 | + it('return true when comment paragraph is active', () => { | |
58 | + helper.component.article = { setting: { comment_paragraph_plugin_activate: true } }; | |
59 | + helper.detectChanges(); | |
60 | + expect(helper.component.isActivated()).toBeTruthy(); | |
61 | + }); | |
62 | + | |
63 | + it('return false when comment paragraph is not active', () => { | |
64 | + expect(helper.component.isActivated()).toBeFalsy(); | |
65 | + }); | |
66 | + | |
67 | + it('return false when article has no setting attribute', () => { | |
68 | + helper.component.article = {}; | |
69 | + helper.detectChanges(); | |
70 | + expect(helper.component.isActivated()).toBeFalsy(); | |
71 | + }); | |
72 | + | |
73 | + it('display activate button when comment paragraph is not active', () => { | |
74 | + expect(helper.all('.comment-paragraph-activate').length).toEqual(1); | |
75 | + expect(helper.all('.comment-paragraph-deactivate').length).toEqual(0); | |
76 | + }); | |
77 | + | |
78 | + it('display deactivate button when comment paragraph is active', () => { | |
79 | + helper.component.article = { setting: { comment_paragraph_plugin_activate: true } }; | |
80 | + helper.detectChanges(); | |
81 | + expect(helper.all('.comment-paragraph-deactivate').length).toEqual(1); | |
82 | + expect(helper.all('.comment-paragraph-activate').length).toEqual(0); | |
83 | + }); | |
84 | + }); | |
85 | +}); | ... | ... |
src/plugins/comment_paragraph/hotspot/comment-paragraph-article-button.component.ts
... | ... | @@ -18,14 +18,19 @@ export class CommentParagraphArticleButtonHotspotComponent { |
18 | 18 | private commentParagraphEventService: CommentParagraphEventService) { } |
19 | 19 | |
20 | 20 | deactivateCommentParagraph() { |
21 | - this.commentParagraphService.deactivateCommentParagraph(this.article).then((result: noosfero.RestResult<noosfero.Article>) => { | |
22 | - this.article = result.data; | |
23 | - this.commentParagraphEventService.toggleCommentParagraph(this.article); | |
24 | - }); | |
21 | + this.toggleCommentParagraph(this.commentParagraphService.deactivateCommentParagraph(this.article)); | |
25 | 22 | } |
26 | 23 | |
27 | 24 | activateCommentParagraph() { |
28 | - this.commentParagraphService.activateCommentParagraph(this.article).then((result: noosfero.RestResult<noosfero.Article>) => { | |
25 | + this.toggleCommentParagraph(this.commentParagraphService.activateCommentParagraph(this.article)); | |
26 | + } | |
27 | + | |
28 | + isActivated() { | |
29 | + return this.article && this.article.setting && this.article.setting.comment_paragraph_plugin_activate; | |
30 | + } | |
31 | + | |
32 | + private toggleCommentParagraph(promise: ng.IPromise<noosfero.RestResult<noosfero.Article>>) { | |
33 | + promise.then((result: noosfero.RestResult<noosfero.Article>) => { | |
29 | 34 | this.article = result.data; |
30 | 35 | this.commentParagraphEventService.toggleCommentParagraph(this.article); |
31 | 36 | }); | ... | ... |
src/plugins/comment_paragraph/http/comment-paragraph.service.spec.ts
0 → 100644
... | ... | @@ -0,0 +1,90 @@ |
1 | +import {CommentParagraphService} from "./comment-paragraph.service"; | |
2 | + | |
3 | + | |
4 | +describe("Services", () => { | |
5 | + | |
6 | + describe("Comment Paragraph Service", () => { | |
7 | + | |
8 | + let $httpBackend: ng.IHttpBackendService; | |
9 | + let commentParagraphService: CommentParagraphService; | |
10 | + | |
11 | + beforeEach(angular.mock.module("noosferoApp", ($translateProvider: angular.translate.ITranslateProvider) => { | |
12 | + $translateProvider.translations('en', {}); | |
13 | + })); | |
14 | + | |
15 | + beforeEach(inject((_$httpBackend_: ng.IHttpBackendService, _CommentParagraphService_: CommentParagraphService) => { | |
16 | + $httpBackend = _$httpBackend_; | |
17 | + commentParagraphService = _CommentParagraphService_; | |
18 | + })); | |
19 | + | |
20 | + | |
21 | + describe("Succesfull requests", () => { | |
22 | + | |
23 | + it("should return paragraph comments by article", (done) => { | |
24 | + let articleId = 1; | |
25 | + $httpBackend.expectGET(`/api/v1/articles/${articleId}/comment_paragraph_plugin/comments?without_reply=true`).respond(200, { comments: [{ body: "comment1" }] }); | |
26 | + commentParagraphService.getByArticle(<noosfero.Article>{ id: articleId }).then((result: noosfero.RestResult<noosfero.Comment[]>) => { | |
27 | + expect(result.data).toEqual([{ body: "comment1" }]); | |
28 | + done(); | |
29 | + }); | |
30 | + $httpBackend.flush(); | |
31 | + }); | |
32 | + | |
33 | + it("should create a paragraph comment", (done) => { | |
34 | + let articleId = 1; | |
35 | + $httpBackend.expectPOST(`/api/v1/articles/${articleId}/comment_paragraph_plugin/comments`).respond(200, { comment: { body: "comment1" } }); | |
36 | + commentParagraphService.createInArticle(<noosfero.Article>{ id: articleId }, <noosfero.Comment>{ body: "comment1" }).then((result: noosfero.RestResult<noosfero.Comment>) => { | |
37 | + expect(result.data).toEqual({ body: "comment1" }); | |
38 | + done(); | |
39 | + }); | |
40 | + $httpBackend.flush(); | |
41 | + }); | |
42 | + | |
43 | + it("activate paragraph comments for an article", (done) => { | |
44 | + let articleId = 1; | |
45 | + $httpBackend.expectPOST(`/api/v1/articles/${articleId}/comment_paragraph_plugin/activate`).respond(200, { article: { title: "article1" } }); | |
46 | + commentParagraphService.activateCommentParagraph(<noosfero.Article>{ id: articleId }).then((result: noosfero.RestResult<noosfero.Article>) => { | |
47 | + expect(result.data).toEqual({ title: "article1" }); | |
48 | + done(); | |
49 | + }); | |
50 | + $httpBackend.flush(); | |
51 | + }); | |
52 | + | |
53 | + it("deactivate paragraph comments for an article", (done) => { | |
54 | + let articleId = 1; | |
55 | + $httpBackend.expectPOST(`/api/v1/articles/${articleId}/comment_paragraph_plugin/deactivate`).respond(200, { article: { title: "article1" } }); | |
56 | + commentParagraphService.deactivateCommentParagraph(<noosfero.Article>{ id: articleId }).then((result: noosfero.RestResult<noosfero.Article>) => { | |
57 | + expect(result.data).toEqual({ title: "article1" }); | |
58 | + done(); | |
59 | + }); | |
60 | + $httpBackend.flush(); | |
61 | + }); | |
62 | + | |
63 | + it("return counts for paragraph comments", (done) => { | |
64 | + let articleId = 1; | |
65 | + $httpBackend.expectGET(`/api/v1/articles/${articleId}/comment_paragraph_plugin/comments/count`).respond(200, { '1': 5, '2': 6 }); | |
66 | + commentParagraphService.commentParagraphCount(<noosfero.Article>{ id: articleId }, '1').then((count: number) => { | |
67 | + expect(count).toEqual(5); | |
68 | + done(); | |
69 | + }); | |
70 | + $httpBackend.flush(); | |
71 | + }); | |
72 | + | |
73 | + it("reset promise when comment paragraph counts fails", (done) => { | |
74 | + let articleId = 1; | |
75 | + commentParagraphService['articleService'] = jasmine.createSpyObj("articleService", ["getElement"]); | |
76 | + commentParagraphService['articleService'].getElement = jasmine.createSpy("getElement").and.returnValue( | |
77 | + { | |
78 | + customGET: (path: string) => { | |
79 | + return Promise.reject({}); | |
80 | + } | |
81 | + } | |
82 | + ); | |
83 | + commentParagraphService.commentParagraphCount(<noosfero.Article>{ id: articleId }, '1').catch(() => { | |
84 | + expect(commentParagraphService['commentParagraphCountsPromise']).toBeNull(); | |
85 | + done(); | |
86 | + }); | |
87 | + }); | |
88 | + }); | |
89 | + }); | |
90 | +}); | ... | ... |
src/plugins/comment_paragraph/side-comments/side-comments.component.spec.ts
0 → 100644
... | ... | @@ -0,0 +1,50 @@ |
1 | +import {SideCommentsComponent} from "./side-comments.component"; | |
2 | +import {ComponentTestHelper, createClass} from '../../../spec/component-test-helper'; | |
3 | +import * as helpers from "../../../spec/helpers"; | |
4 | +import {Provider} from 'ng-forward'; | |
5 | +import {ComponentFixture} from 'ng-forward/cjs/testing/test-component-builder'; | |
6 | + | |
7 | +let htmlTemplate = '<comment-paragraph-side-comments [article]="ctrl.article" [paragraph-uuid]="ctrl.paragraphUuid"></comment-paragraph-side-comments>'; | |
8 | + | |
9 | +describe("Components", () => { | |
10 | + describe("Side Comments Component", () => { | |
11 | + | |
12 | + let serviceMock = jasmine.createSpyObj("CommentParagraphService", ["getByArticle"]); | |
13 | + serviceMock.getByArticle = jasmine.createSpy("getByArticle").and.returnValue(Promise.resolve({ data: {} })); | |
14 | + | |
15 | + let commentServiceMock = {}; | |
16 | + let postCommentEventService = jasmine.createSpyObj("postCommentEventService", ["emit", "subscribe"]); | |
17 | + postCommentEventService.subscribe = jasmine.createSpy("subscribe"); | |
18 | + | |
19 | + let providers = [ | |
20 | + new Provider('CommentParagraphService', { useValue: serviceMock }), | |
21 | + new Provider('CommentService', { useValue: commentServiceMock }), | |
22 | + new Provider('PostCommentEventService', { useValue: postCommentEventService }) | |
23 | + ]; | |
24 | + let helper: ComponentTestHelper; | |
25 | + | |
26 | + beforeEach(angular.mock.module("templates")); | |
27 | + | |
28 | + beforeEach((done) => { | |
29 | + let cls = createClass({ | |
30 | + template: htmlTemplate, | |
31 | + directives: [SideCommentsComponent], | |
32 | + providers: providers, | |
33 | + properties: { | |
34 | + paragraphUuid: "uuid", | |
35 | + article: {} | |
36 | + } | |
37 | + }); | |
38 | + helper = new ComponentTestHelper(cls, done); | |
39 | + }); | |
40 | + | |
41 | + it('call service to load paragraph comments', () => { | |
42 | + helper.component.loadComments(); | |
43 | + expect(serviceMock.getByArticle).toHaveBeenCalled(); | |
44 | + }); | |
45 | + | |
46 | + it('set paragraph uuid in new comment object', () => { | |
47 | + expect(helper.component.newComment['paragraph_uuid']).toEqual('uuid'); | |
48 | + }); | |
49 | + }); | |
50 | +}); | ... | ... |
src/plugins/comment_paragraph/side-comments/side-comments.component.ts
... | ... | @@ -2,19 +2,23 @@ import {Component, Inject, Input, Output} from "ng-forward"; |
2 | 2 | import {CommentsComponent} from "../../../app/article/comment/comments.component"; |
3 | 3 | import {CommentService} from "../../../lib/ng-noosfero-api/http/comment.service"; |
4 | 4 | import {CommentParagraphService} from "../http/comment-paragraph.service"; |
5 | +import { PostCommentEventService } from "../../../app/article/comment/post-comment/post-comment-event.service"; | |
5 | 6 | |
6 | 7 | @Component({ |
7 | 8 | selector: "comment-paragraph-side-comments", |
8 | 9 | templateUrl: 'app/article/comment/comments.html', |
9 | 10 | }) |
10 | -@Inject(CommentService, "$rootScope", CommentParagraphService) | |
11 | +@Inject(CommentService, PostCommentEventService, "$scope", CommentParagraphService) | |
11 | 12 | export class SideCommentsComponent extends CommentsComponent { |
12 | 13 | |
13 | 14 | @Input() article: noosfero.Article; |
14 | 15 | @Input() paragraphUuid: string; |
15 | 16 | |
16 | - constructor(commentService: CommentService, $rootScope: ng.IScope, private commentParagraphService: CommentParagraphService) { | |
17 | - super(commentService, $rootScope); | |
17 | + constructor(commentService: CommentService, | |
18 | + postCommentEventService: PostCommentEventService, | |
19 | + $scope: ng.IScope, | |
20 | + private commentParagraphService: CommentParagraphService) { | |
21 | + super(commentService, postCommentEventService, $scope); | |
18 | 22 | } |
19 | 23 | |
20 | 24 | ngOnInit() { | ... | ... |
src/spec/component-test-helper.ts
... | ... | @@ -68,6 +68,8 @@ export class ComponentTestHelper<T extends any> { |
68 | 68 | this.init(done); |
69 | 69 | } |
70 | 70 | |
71 | + fixture: any; | |
72 | + | |
71 | 73 | /** |
72 | 74 | * @ngdoc method |
73 | 75 | * @name init |
... | ... | @@ -79,7 +81,8 @@ export class ComponentTestHelper<T extends any> { |
79 | 81 | let promisse = this.tcb.createAsync(this.mockComponent) as Promise<ComponentFixture>; |
80 | 82 | return promisse.then((fixture: any) => { |
81 | 83 | // Fire all angular events and parsing |
82 | - fixture.detectChanges(); | |
84 | + this.fixture = fixture; | |
85 | + this.detectChanges(); | |
83 | 86 | // The main debug element |
84 | 87 | this.debugElement = fixture.debugElement; |
85 | 88 | this.component = <T>this.debugElement.componentViewChildren[0].componentInstance; |
... | ... | @@ -96,6 +99,17 @@ export class ComponentTestHelper<T extends any> { |
96 | 99 | |
97 | 100 | /** |
98 | 101 | * @ngdoc method |
102 | + * @name detectChanges | |
103 | + * @methodOf spec.ComponentTestHelper | |
104 | + * @description | |
105 | + * Detect changes in component | |
106 | + */ | |
107 | + detectChanges() { | |
108 | + this.fixture.detectChanges(); | |
109 | + } | |
110 | + | |
111 | + /** | |
112 | + * @ngdoc method | |
99 | 113 | * @name all |
100 | 114 | * @methodOf spec.ComponentTestHelper |
101 | 115 | * @description | ... | ... |